Basics Git usage with conflict resolution steps
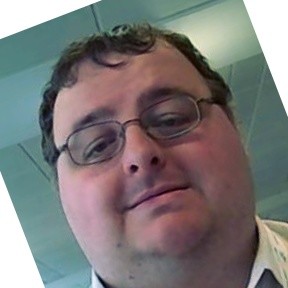
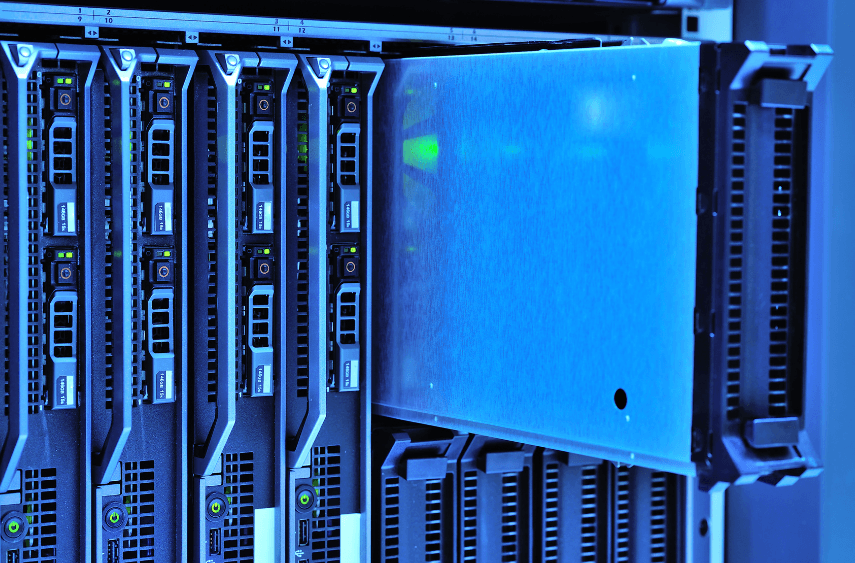
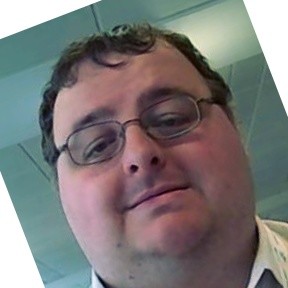
Step-by-Step Guide for Using Git (Level 100)
1. Install Git
Ensure Git is installed on your system. If it's not installed, download and install it from here. Like I said basic, also make sure you powered on your laptop today...
2. Initialize a Git Repository
Navigate to your project directory and initialize a Git repository.
cd path/to/your//project
git init
3. Create a .gitignore File
Create a .gitignore file to exclude files that do not need to be version controlled, such as temporary files or build outputs.
touch .gitignore
Add the following content to the .gitignore file:
*.tmp
*.cache
output/
# System files
.DS_Store
Thumbs.db
4. Add and Commit Your Project Files
Add your project files to the repository and commit them.
git add .
git commit -m "Initial commit - Add project files"
5. Create a New Branch
Create a new branch for your work. This helps in managing different versions or features of your project.
git checkout -b my-feature-branch
6. Regularly Commit Changes
As you make changes to your project, regularly commit those changes.
git add .
git commit -m "Describe your changes"
7. Push to a Remote Repository
If you have a remote repository (e.g., on GitHub, GitLab, or Bitbucket), you can push your commits to the remote repository.
git remote add origin https://github.com/yourusername/your-repo.git
git push -u origin my-feature-branch
8. Collaborate with Others
If working in a team, you can pull changes from the remote repository and merge them into your branch.
git pull origin main
git merge main
9. Resolve Conflicts
When multiple people are working on the same project, or when you are working on different branches and trying to merge them, conflicts can occur if changes have been made to the same parts of the code or files. Here's how to handle those conflicts:
9.1 Pull Changes from Remote
When you try to pull changes from a remote branch or merge another branch into your current branch, Git will attempt to merge the changes. If it encounters conflicts, it will stop and mark the conflicted files.
git pull origin main
or
git merge main
9.2 Identify Conflicted Files
Git will list the files that have conflicts. You can also see the status of conflicted files using:
git status
Conflicted files will be marked as "both modified."
9.3 Open and Edit Conflicted Files
Open each conflicted file in your text editor. Git marks conflicts in the files using special markers:
<<<<<<< HEAD
Your changes
=======
Changes from the branch you are merging
>>>>>>> branch-name
<<<<<<< HEAD: This marks the start of the section with your changes.
=======: This separates your changes from the changes in the branch you are merging.
>>>>>>> branch-name: This marks the end of the conflicting changes from the branch you are merging.
9.4 Resolve the Conflict
You need to decide how to resolve the conflict. You can choose to keep your changes, the other branch's changes, or a combination of both. After making your decision, remove the conflict markers (<<<<<<<, =======, and >>>>>>>) and edit the file accordingly.
For example, if you have the following conflict:
<<<<<<< HEAD
print("Hello from your changes")
=======
print("Hello from the branch you are merging")
>>>>>>> branch-name
You can resolve it like this:
print("Hello from both changes")
9.5 Add the Resolved Files
Once you have resolved the conflicts, you need to add the resolved files to the staging area:
git add <file-name>
Do this for each conflicted file you have resolved.
9.6 Commit the Resolved Changes
After all conflicts are resolved and added to the staging area, commit the changes:
git commit -m "Resolve merge conflicts"
This creates a new commit with the resolved changes.
Example Walkthrough Let's say you are on a branch called feature-branch, and you want to merge changes from main.
Attempt to Merge
git checkout feature-branch
git merge main
Git Detects a Conflict Git outputs something like:
Auto-merging file.txt
CONFLICT (content): Merge conflict in file.txt
Automatic merge failed; fix conflicts and then commit the result.
Check Status
git status
Output:
On branch feature-branch
You have unmerged paths.
(fix conflicts and run "git commit")
Unmerged paths:
(use "git add <file>..." to mark resolution)
both modified: file.txt
Open the Conflicted File
In file.txt, you see:
<<<<<<< HEAD
Line from feature-branch
=======
Line from main
>>>>>>> main
Resolve the Conflict
Edit the file to resolve the conflict: Then Add and Commit
git add file.txt
git commit -m "Resolve merge conflicts in file.txt"
10. Switch Between Branches
Switch between branches as needed to work on different features or versions.
git checkout main
git pull origin main
Example Script for Automation
You can create a script (e.g., git_.sh) to automate some of these steps. Below is a basic example:
#!/bin/bash
# Variables
REPO_URL="https://github.com/yourusername/your-repo.git"
BRANCH_NAME="my-feature-branch"
# Initialize Git repository
git init
# Create .gitignore if it doesn't exist
if [ ! -f .gitignore ]; then
echo -e "*.tmp\n*.cache\noutput/\n.DS_Store\nThumbs.db" > .gitignore
fi
# Add and commit files
git add .
git commit -m "Initial commit - Add project files"
# Create new branch
git checkout -b $BRANCH_NAME
# Add remote repository
git remote add origin $REPO_URL
# Push to remote repository
git push -u origin $BRANCH_NAME
echo "Git repository setup completed. You are on branch $BRANCH_NAME"
Make the script executable and run it:
chmod +x git_.sh
./git_.sh
This script initializes a Git repository, creates a .gitignore file, commits the initial files, creates a new branch, and pushes the branch to the specified remote repository.
Conclusion
By following these steps and using the script, you can effectively manage your project files with Git, enabling version control, collaboration, and better project management.