Flask API on ECS and Lambda
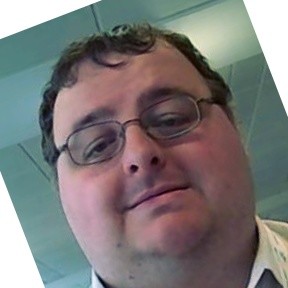
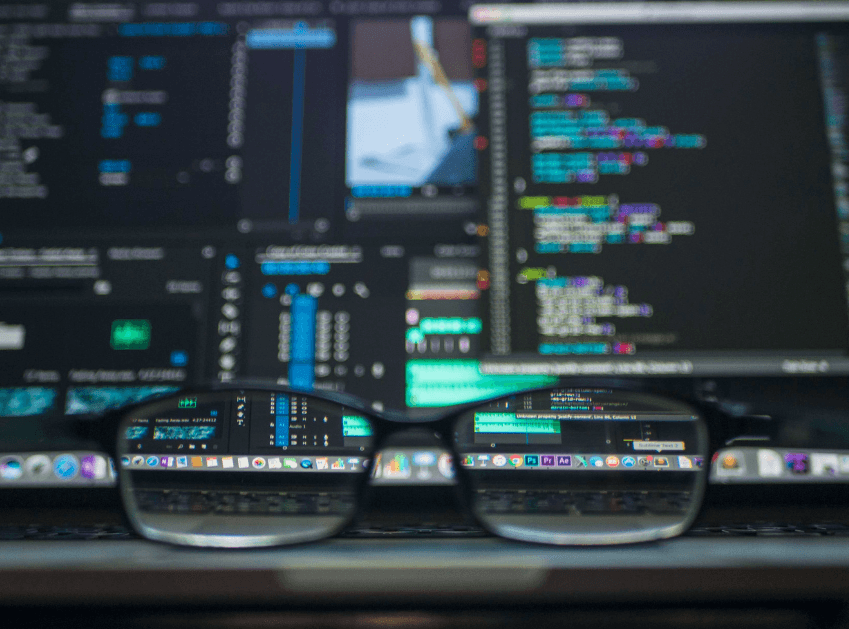
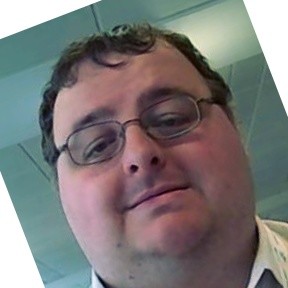
Deploying a Flask API to AWS ECS and AWS Lambda
Introduction
Flask is a popular micro web framework for building APIs in Python. Deploying Flask applications to the cloud can be done using various services, but two of the most commonly used services in AWS for deploying APIs are Elastic Container Service (ECS) and AWS Lambda. This post will walk you through the steps for deploying a Flask API to both ECS and Lambda.
Prerequisites
Before we start, ensure you have the following:
- AWS account
- AWS CLI installed and configured
- Docker installed (for ECS)
- Python installed
- Deploying Flask API to AWS ECS
- Step 1: Create a Flask Application
- Create a simple Flask application:
# app.py
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/')
def home():
return jsonify(message="Hello, world!")
if __name__ == "__main__":
app.run(host='0.0.0.0', port=5000)
Step 2: Create a Dockerfile
Create a Dockerfile to containerize the Flask application:
Copy code
# Dockerfile
FROM python:3.8-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Create a requirements.txt file:
Flask==2.0.1
Step 3: Build and Push the Docker Image
Build the Docker image:
docker build -t flask-api .
Tag the image:
docker tag flask-api:latest YOUR_ECR_URI/flask-api:latest
Push the image to Amazon ECR:
Copy code
docker push YOUR_ECR_URI/flask-api:latest
Step 4: Create ECS Cluster and Task Definition
Create ECS Cluster:
Go to the ECS console and create a new cluster. Create Task Definition:
Define a new task with the Docker image URI and necessary configurations.
Step 5: Create Service
Create a service that uses the task definition. Configure it to use the desired number of tasks and set up auto-scaling if needed.
Step 6: Configure Load Balancer (Optional)
To expose your API, you can set up an Application Load Balancer (ALB) and configure it to route traffic to your ECS service.
Deploying Flask API to AWS Lambda
Step 1: Create a Flask Application
Use the same Flask application as before.
Step 2: Create a Lambda Function
Install Zappa:
Copy code
pip install zappa
Initialize Zappa:
Copy code
zappa init
This will create a zappa_settings.json file. Configure it as needed.
Deploy with Zappa:
Copy code
zappa deploy
Step 3: Update Lambda Function
To update the function after making changes:
Copy code
zappa update
Comparison: ECS vs. Lambda
ECS:
Pros:
- Suitable for long-running applications.
- Full control over the environment.
- Easy to scale with ECS auto-scaling.
Cons:
- Requires managing EC2 instances or Fargate tasks.
- Higher complexity and cost for small workloads.
Lambda:
Pros:
- Ideal for short-lived, event-driven applications.
- Automatic scaling and pay-per-use model.
- No need to manage servers.
Cons:
- Cold start latency.
- Limited execution time (15 minutes maximum).
Conclusion
Both ECS and Lambda are powerful options for deploying Flask APIs on AWS, each with its own strengths and use cases. ECS provides more control and is better for long-running applications, while Lambda offers simplicity and cost-efficiency for event-driven workloads. By understanding the differences and following the steps outlined above, you can deploy your Flask API using the method that best fits your needs.
Code for Flask and terraform to deploy something along these lines can be found here : Github